image-to-ascii
A python program to convert an image into an ascii image
Introduction
This program allows you to convert images into ASCII art. It uses various parameters to control the conversion process, including image scaling, sharpness adjustment, brightness adjustment, character set, and more.
Installation
- Clone this repository to your local machine.
git clone https://www.github.com/ajratnam/image-to-ascii.git
- Activate the poetry environment.
poetry shell
- Install the dependencies.
poetry install
Usage
The documentation for the program can be read here.
Examples
Here are some examples of how to use the program:
Convert an image to ASCII art.
from converter import image_to_ascii
print(image_to_ascii('images/angry_bird.jpg'))
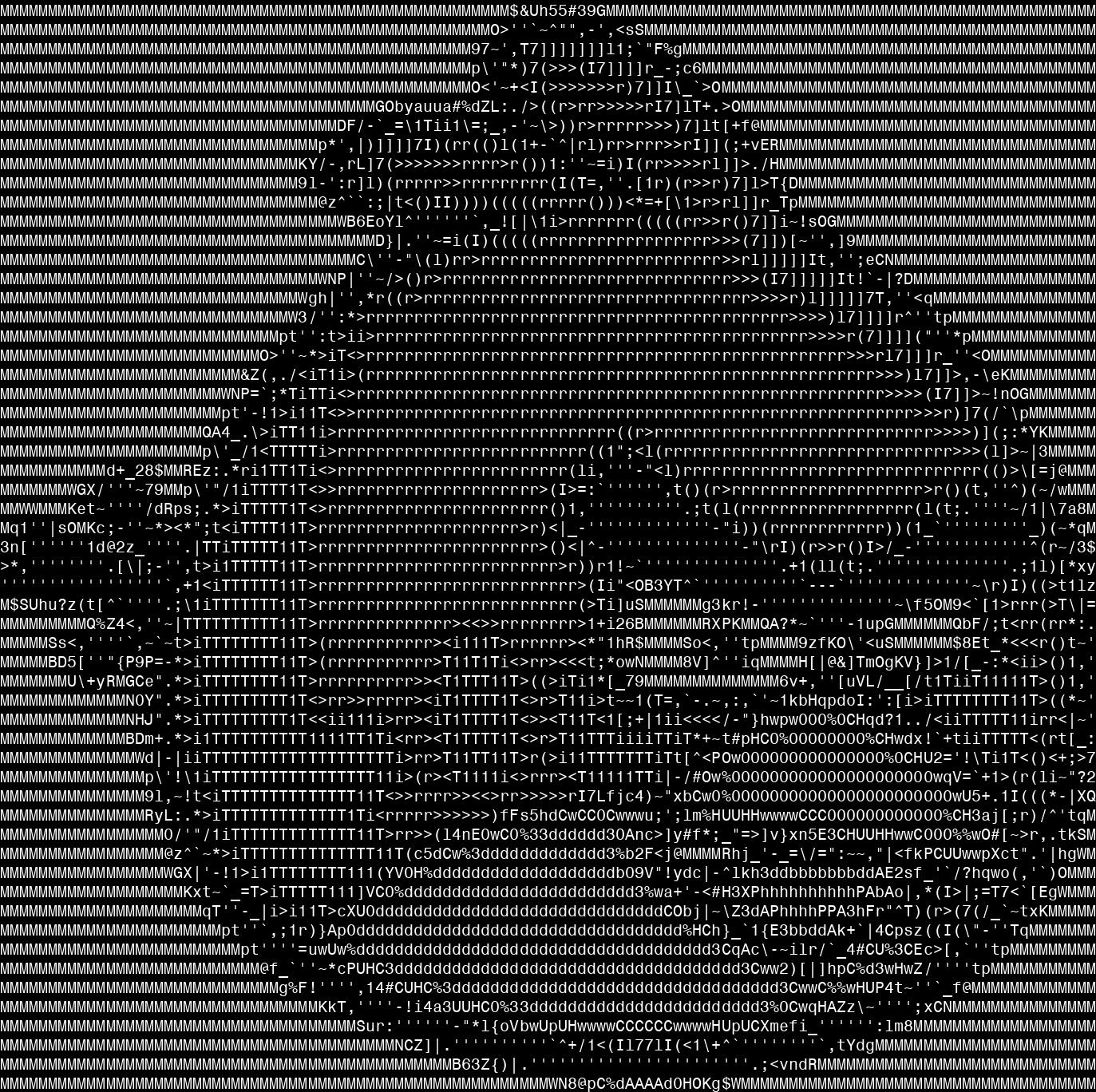
Save the ASCII art to an image.
from converter import image_to_ascii, ascii_to_image
ascii_art = image_to_ascii('images/angry_bird.jpg')
ascii_art_image = ascii_to_image(ascii_art)
ascii_art_image.save('images/angry_bird_ascii.jpg')
Change the size of the ascii art by absolute pixels.
from converter import image_to_ascii
print(image_to_ascii("images/angry_bird.jpg", size=(30, 30)))
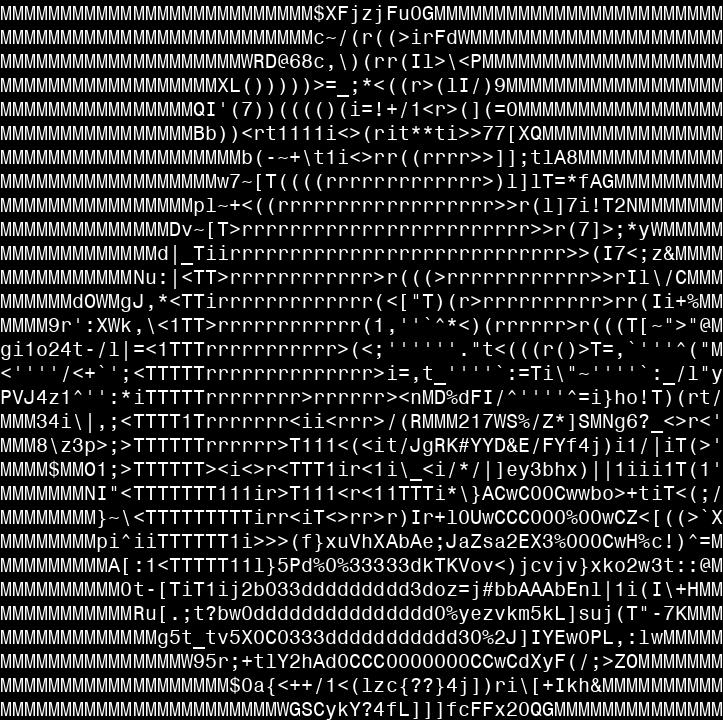
Change the size of the ascii art by absolute pixels but do not try to maintain visual aspect ratio.
from converter import image_to_ascii
print(image_to_ascii("images/angry_bird.jpg", size=(30, 30), fix_scaling=False))
Change the size of the ascii art by relative scaling.
from converter import image_to_ascii
print(image_to_ascii("images/angry_bird.jpg", scale=0.5))
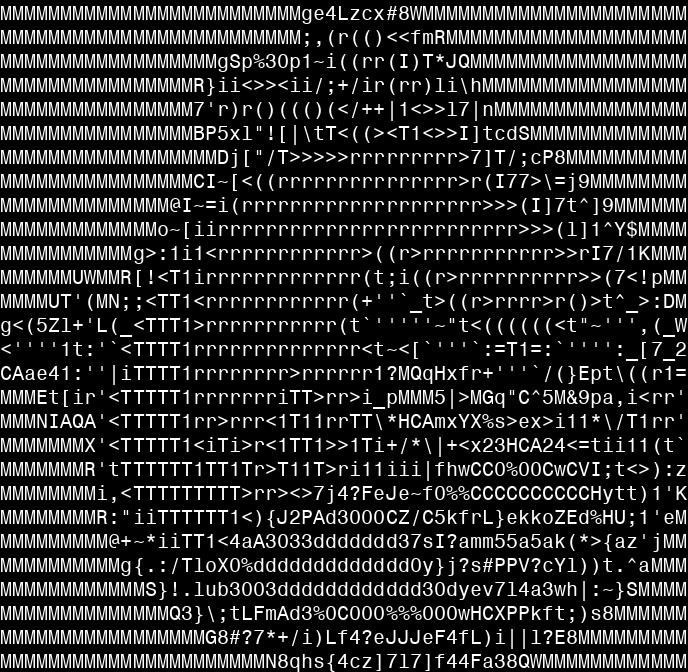
Brighten the ascii art.
from converter import image_to_ascii
print(image_to_ascii("images/angry_bird.jpg", brightness=3))
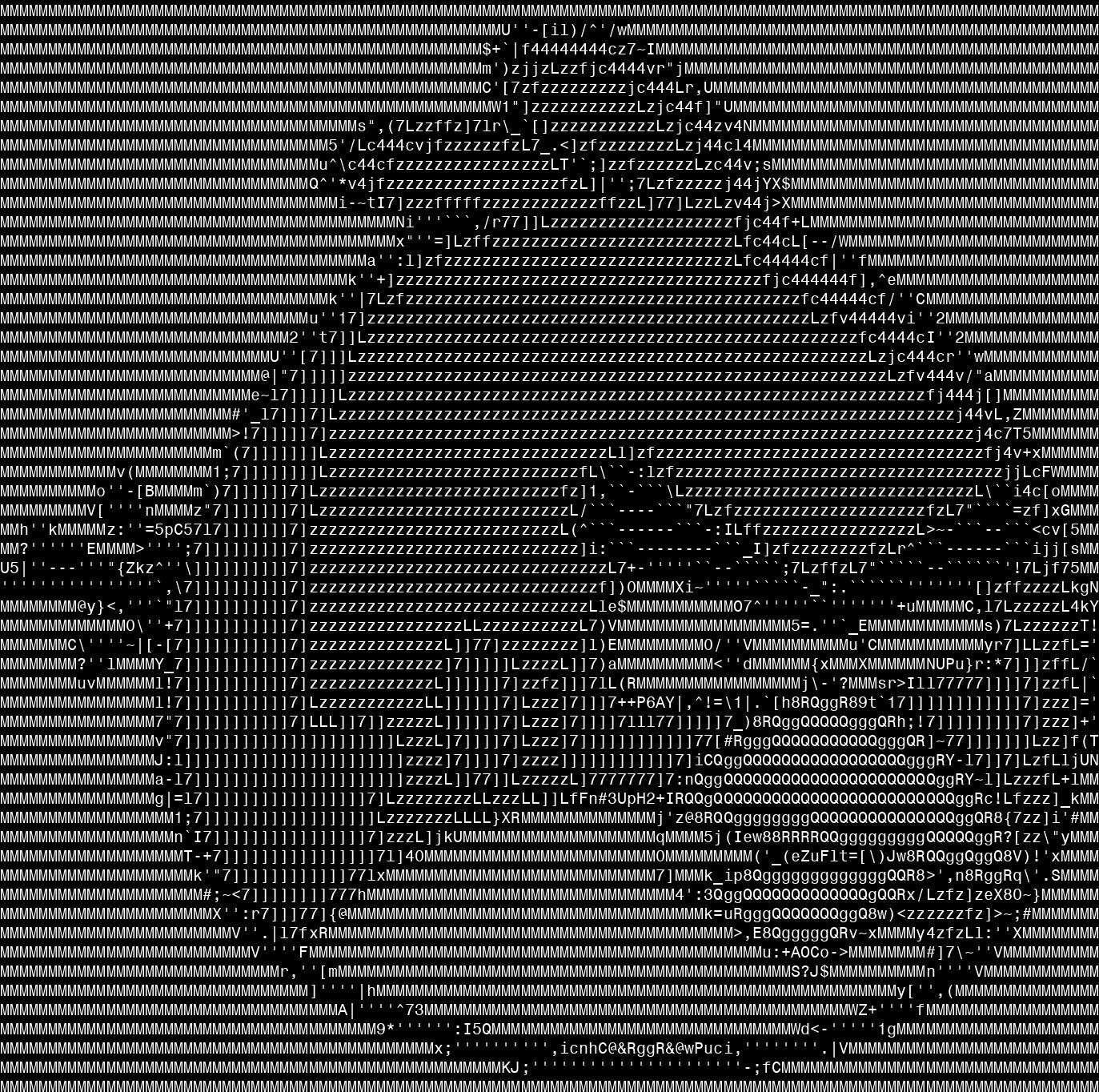
Darken the ascii art.
from converter import image_to_ascii
print(image_to_ascii("images/angry_bird.jpg", brightness=0.2))
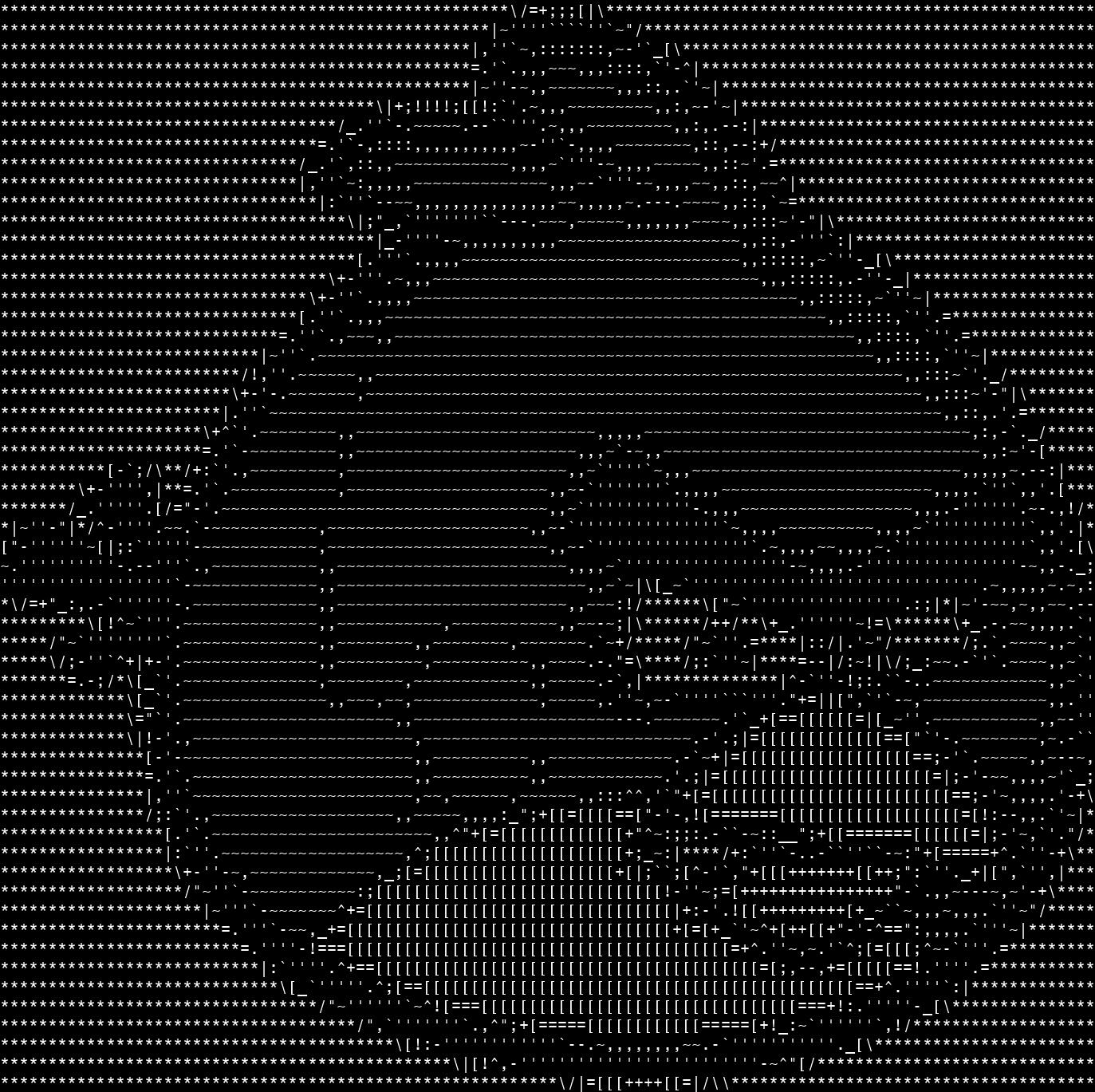
Sharpen the ascii art.
from converter import image_to_ascii
print(image_to_ascii("images/angry_bird.jpg", sharpness=3))
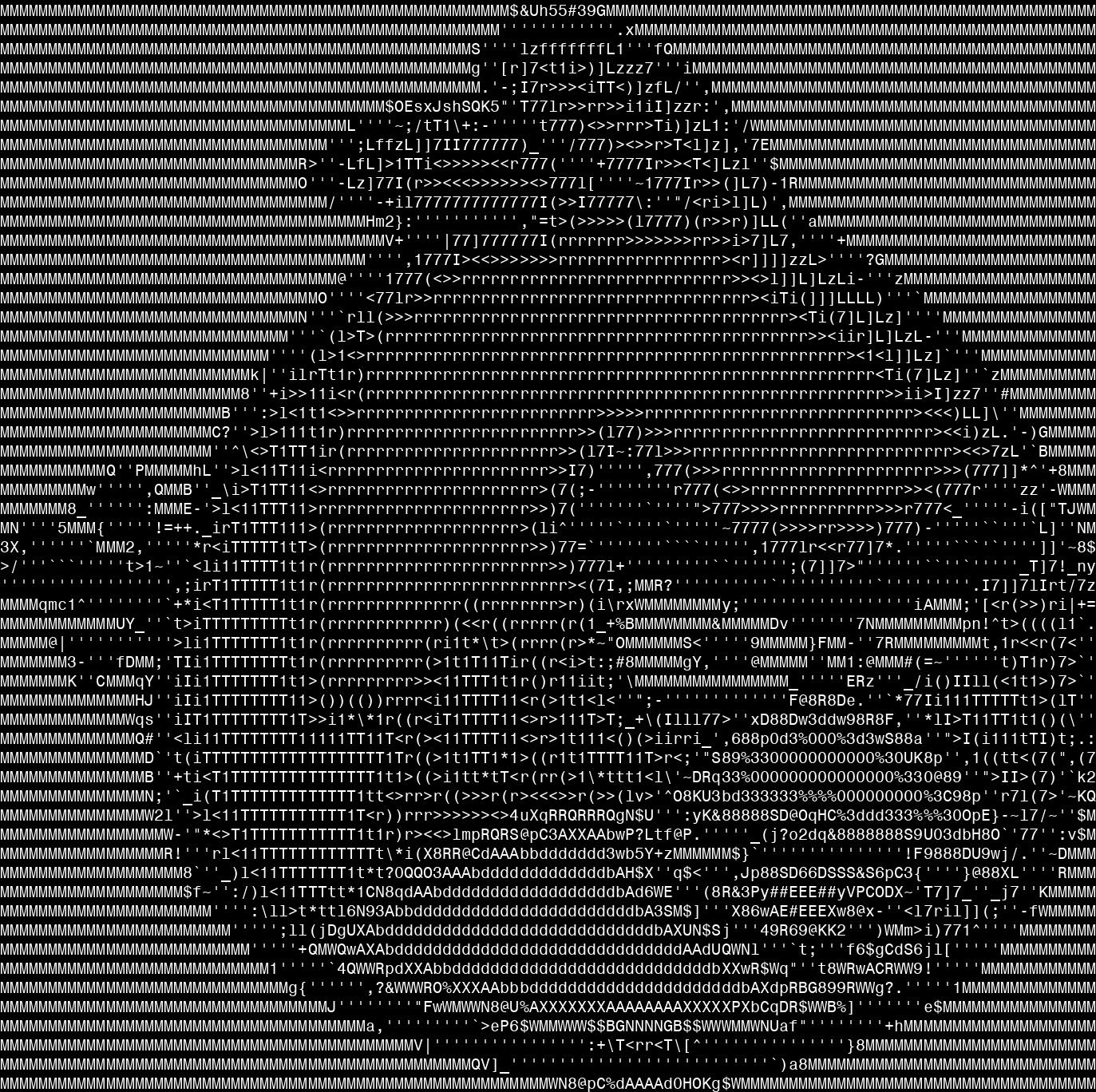
Blur the ascii art.
from converter import image_to_ascii
print(image_to_ascii("images/angry_bird.jpg", sharpness=0.2))
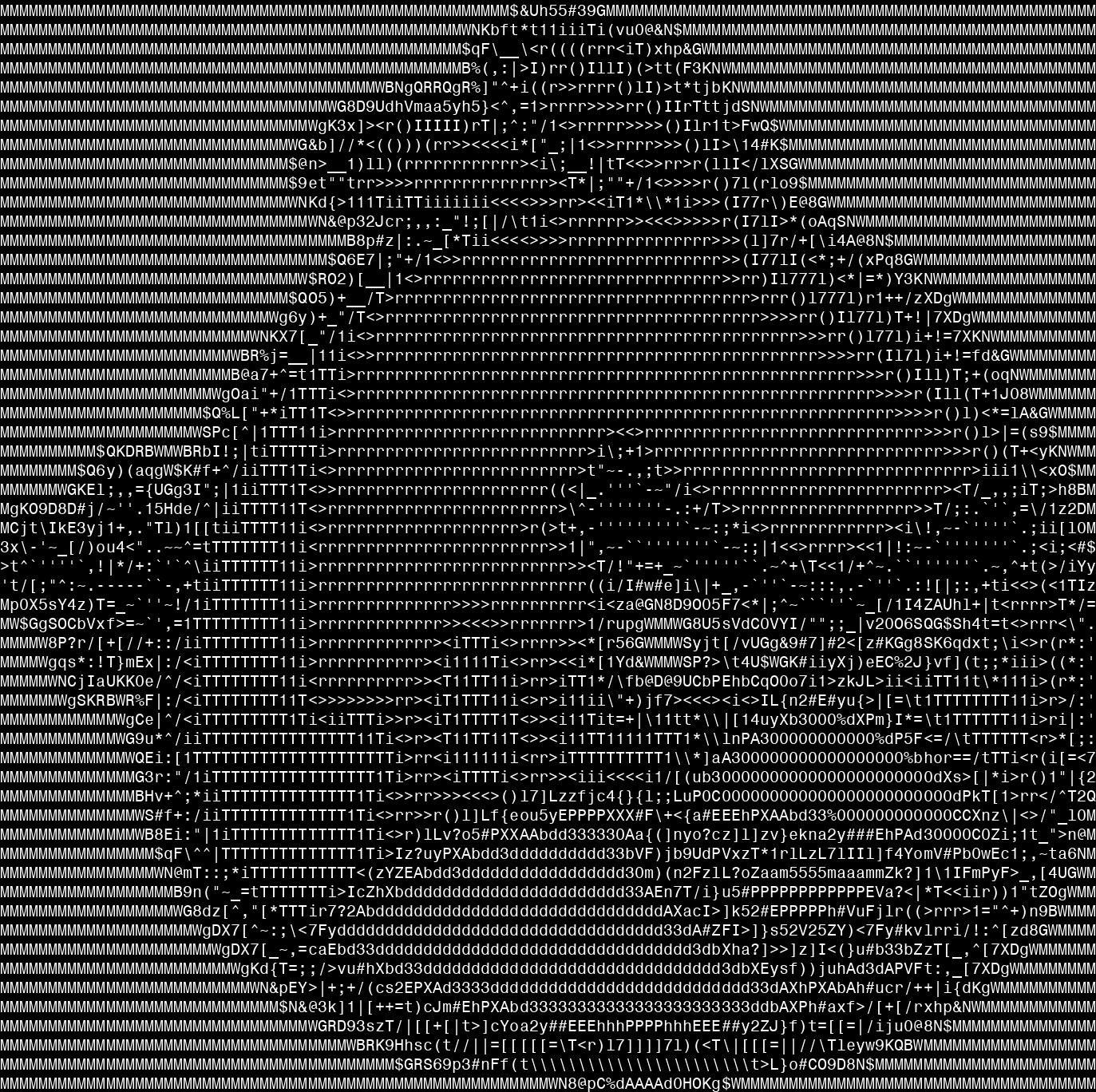
Change the character set used to generate the ascii art.
from converter import image_to_ascii
print(image_to_ascii("images/angry_bird.jpg", charset=' ░▒▓█'))
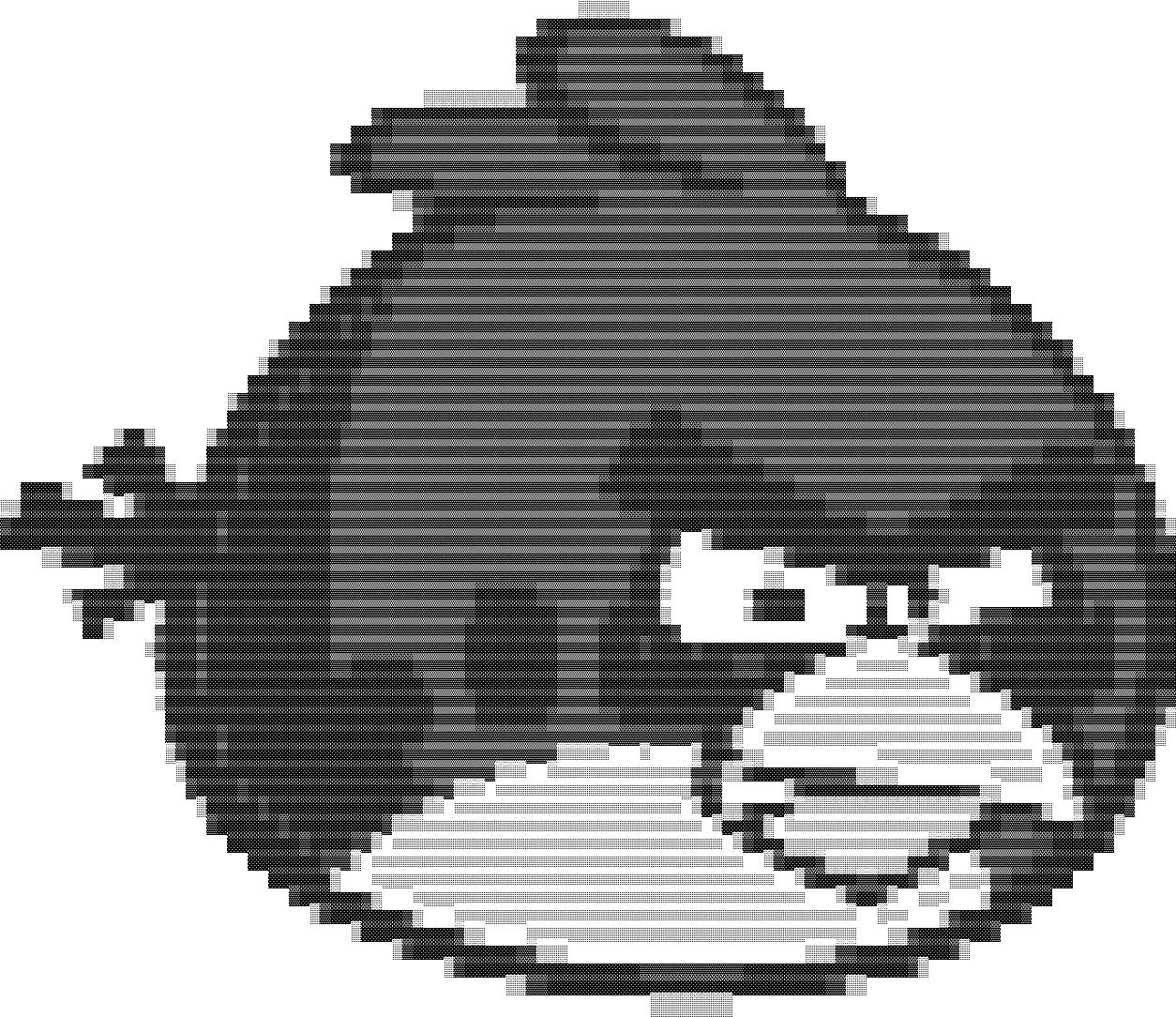
Print colored ascii art to the console.
from converter import image_to_ascii
print(image_to_ascii("images/angry_bird.jpg", colorful=True))
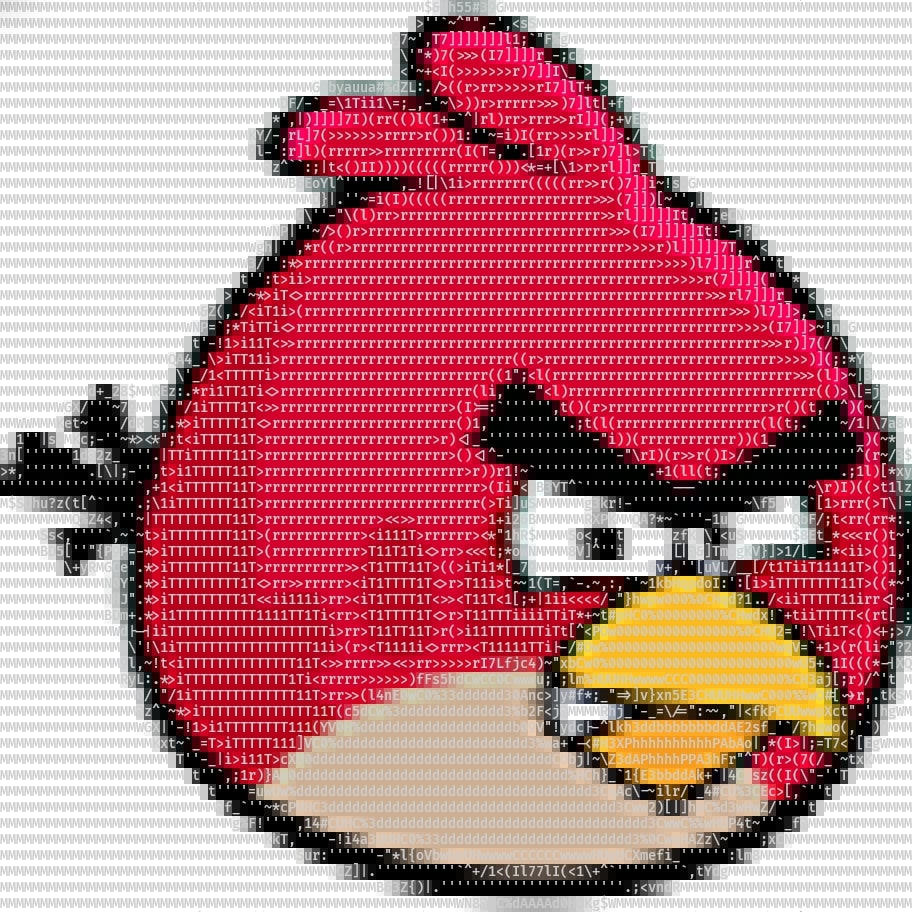
License
This project is licensed under the MIT License.
Contributing
Contributions are welcome! If you have any suggestions or improvements, feel free to create an issue or a pull request.
Install the additional development dependencies with poetry install --dev
.
Run coverage test with python -m pytest --cov